일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 |
- SQL
- 봤어요처리
- svn
- db
- ojdbc6
- datagrip 한글깨짐
- tecoble
- eqauls-hashcode
- javascript error
- JavaScript
- Aspect
- InteliJ
- 코어자바스크립트
- MySQL
- Mac
- @RequestBody
- Spring
- REST
- TypeScript
- oracle
- 프로그래머스
- Java
- 프로젝트 여러 개
- node.js
- class-transformer
- maven
- Stream
- DART
- kubernetes dns 질의
- 인텔리제이
- Today
- Total
개발자가 되고 싶은 개발자
JavaScript의 기본 Error 객체 본문
Error() constructor - JavaScript | MDN
The Error() constructor creates Error objects.
developer.mozilla.org
TL;DR
자바스크립트에 내장된 기본 Error 객체는 name, message 파라미터를 받는다
에러를 반환할 때 호출되는 toString 메소드는 name이 존재하지 않을 경우 "Error: "와 message를 붙여서 반환하게 된다.
일반적인 에러 관리법
보통 에러를 반환할 때는, 기본 에러 타입을 상속받아서 커스텀한 메시지를 반환하는 형태를 이용합니다.
interface HttpError {
status?: number;
message?: string;
code?: number;
}
interface HttpErrors {
errors: HttpError[];
}
declare class HttpException extends Error {
status: number;
message: string;
errors: HttpError | HttpErrors;
constructor(status: number, message: string, code?: number, errors?: any);
json(): HttpError | HttpErrors;
}
export default HttpException;
export class CustomException extends HttpException {
constructor(message: string = "Someting text…") {
super(HttpStatusCode.SERVER_ERROR_500, message);
this.name = "CustomException";
}}
}
커스텀 메시지
어떠한 에러가 발생했는지에 대한 내용을 표현하는 message에는 클라이언트와 협의하여 방식을 채택하고 있습니다.
- 서버에서 반환한 메시지를 그대로 보여주거나
- 사전에 협의한 code 값으로 프론트에서 처리하거나
- 2번과 같이 한다면 message는 디버깅용에 사용하곤 합니다.
정리하는 이유
문제가 되었던 부분은 다음과 같습니다,
Error: 서버에 문제가 발생했습니다. 관리자에게 문의해주세요.
기획에서는 ”Error:“ 텍스트를 요청한 적이 없었고, 코드 에디터에서 별도로 해당 텍스트를 검색해봐도 작성한 부분이 없었습니다.
20.5.3.3 Error.prototype.name
ECMAScript® 2025 Language Specification
Introduction This Ecma Standard defines the ECMAScript 2025 Language. It is the sixteenth edition of the ECMAScript Language Specification. Since publication of the first edition in 1997, ECMAScript has grown to be one of the world's most widely used gener
tc39.es
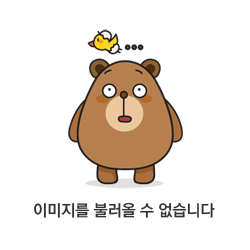
명세에 따르면 name이 없으면 초기 값은 “Error”라고 명시되어 있습니다.
9번에 따르면 콜론과 스페이스를 덧붙이는 것을 알 수 있습니다.
명세서에는 코드가 나와있지 않지만 위 명세를 예시로 들면 아래와 같습니다.
Error.prototype.toString = function() {
let name = this.name !== undefined ? String(this.name) : "Error";
let msg = this.message !== undefined ? String(this.message) : "";
if (name === "") return msg;
if (msg === "") return name;
return `${name}: ${msg}`;
};
몰랐던 부분에 대해서 정리합니다.
'Dev > JavaScript & TypeScript' 카테고리의 다른 글
class-validator 사용법 (0) | 2024.08.15 |
---|---|
class-transformer란 무엇이고 왜 사용할까? (0) | 2024.08.15 |
JavaScript의 Number 표현범위 (Java의 int를 곁들인…) (0) | 2024.07.18 |
시퀄라이즈의 타입스크립트 호환성 (0) | 2024.07.03 |
인프런 강의 안봤는데 봤어요 스크립트 (3) | 2024.06.28 |